Using Cloudflare Workers to Filter Traffic by Country and Secure Your API Endpoint
Cloudflare Workers is a serverless platform that allows developers to run their code on the edge of the Cloudflare network. One of the many use cases for Cloudflare Workers is blocking countries from accessing a specific API endpoint. This can be useful for a variety of reasons, such as compliance with local laws, mitigating attacks from certain regions, or reducing server load.
In this article, we will go over how to use Cloudflare Workers to block countries from accessing a specific API endpoint, using the built-in cf-ipcountry
header to determine the country of the user making the request.
Step 1: Create a Cloudflare Worker
To get started, log in to your Cloudflare account and navigate to the Workers section. Click on the "Create a Worker" button to start a new Worker.
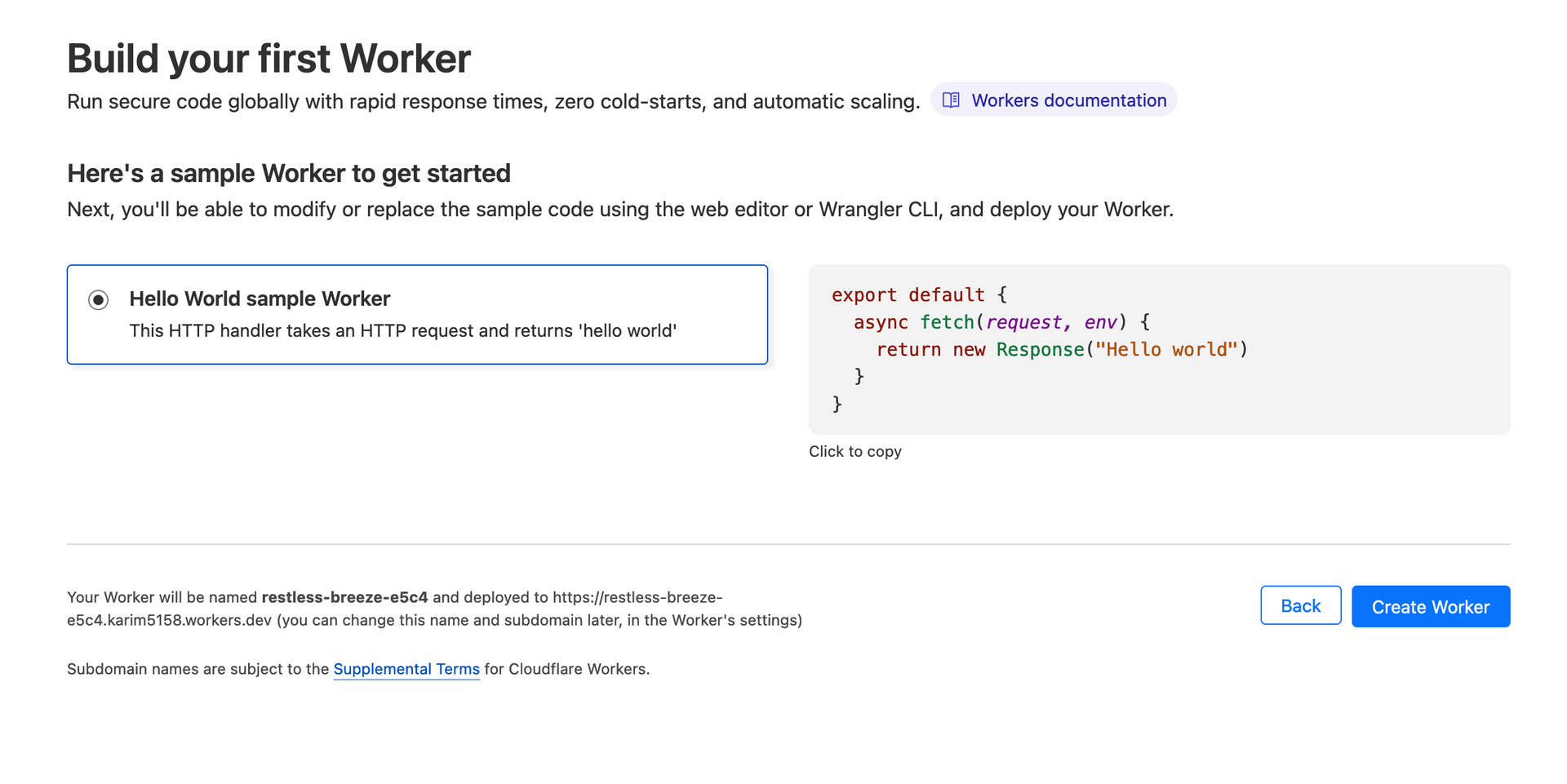
You will be redirected to the worker editor
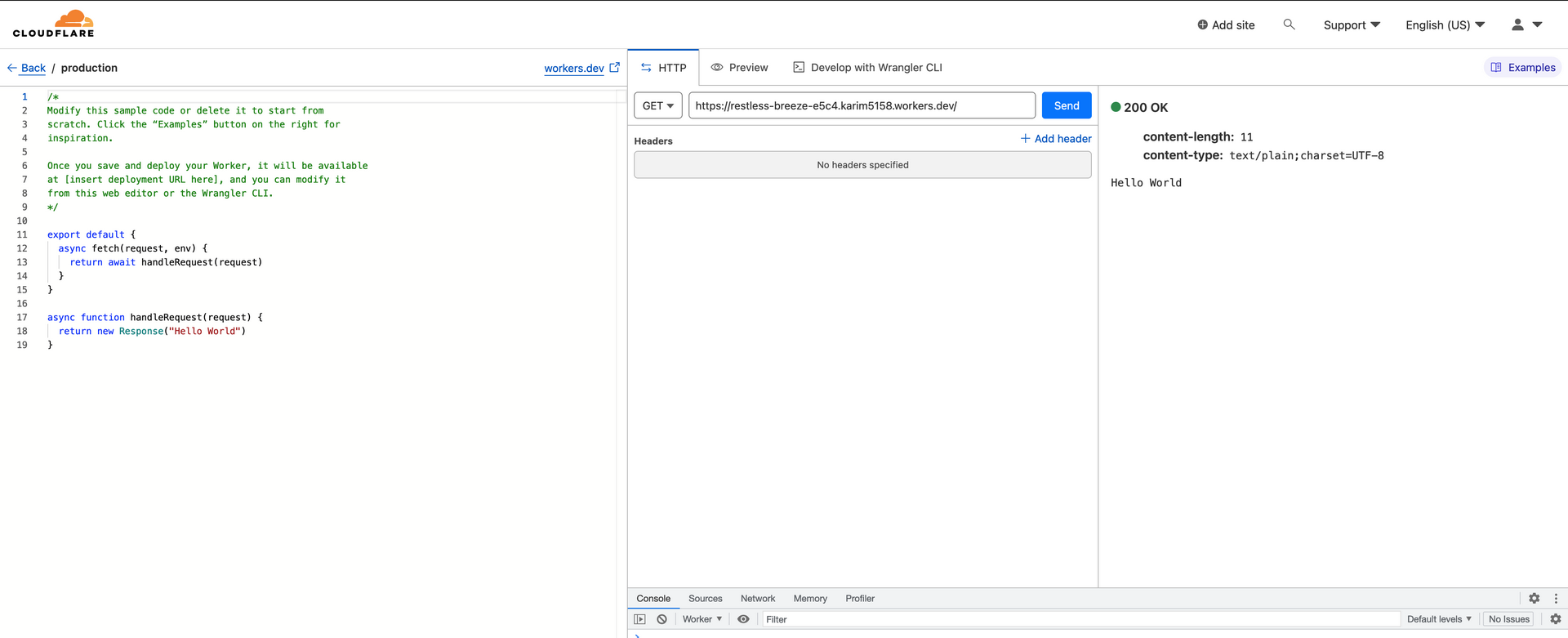
Paste in the following code:
export default {
async fetch(request) {
// An array of allowed countries
const allowedCountries = ["GR", "MA", "LB"]
// Get the country code from the incoming request headers
const country = request.headers.get("cf-ipcountry");
// If the country code is not in the allowedCountries array
if (!allowedCountries.includes(country)) {
// Return a response with a "403 Forbidden" status code
return new Response("Not allowed", { status: 403 });
}
// If the country is allowed, fetch and return the original request
return fetch(request);
}
}
This code sets up a Cloudflare Worker that intercepts all incoming requests to your website. It checks the country of the user making the request using the built-in cf-ipcountry
header. If the country is not on the list of allowed countries, it returns a "403 Not allowed" response. If the country is allowed, it allows the request to proceed to your API endpoint.
Define the Allowed Countries
In the code above, you need to replace ["GR", "MA", "LB"] with an array of the two-letter ISO country codes of the countries you want to allow. You can find a list of country codes on the ISO website.
Step 2: Deploy the Cloudflare Worker
Once you have customized the code to your needs, click the "Save and Deploy" button to deploy the Cloudflare Worker.
To rename your worker click on "Manage Service"
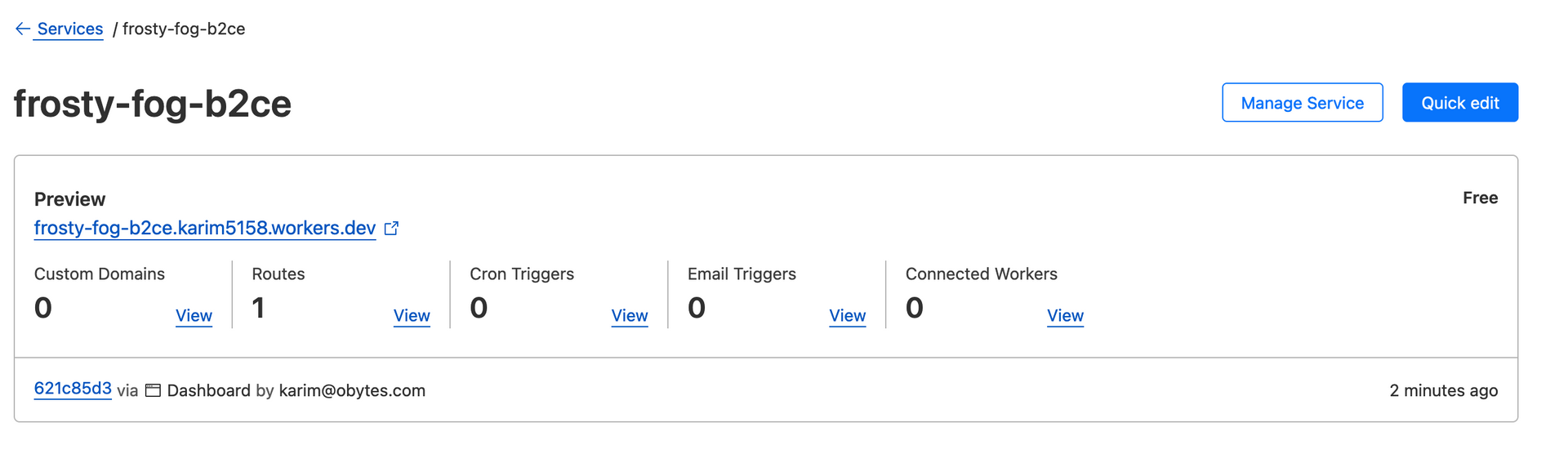
Step 3: Set Up a Trigger for Your Cloudflare Worker
A trigger in Cloudflare Workers is a configurable mechanism that specifies the precise time or situation in which a worker script should be activated. Specifically, the worker script will be triggered to execute when incoming traffic reaches a predetermined endpoint in our case.
To set up a trigger for your Cloudflare Worker, you need to navigate to the "Triggers" tab in the Workers section of your Cloudflare account. Click the "Add Route" button to create a new route. You can specify the route and zone for your route.
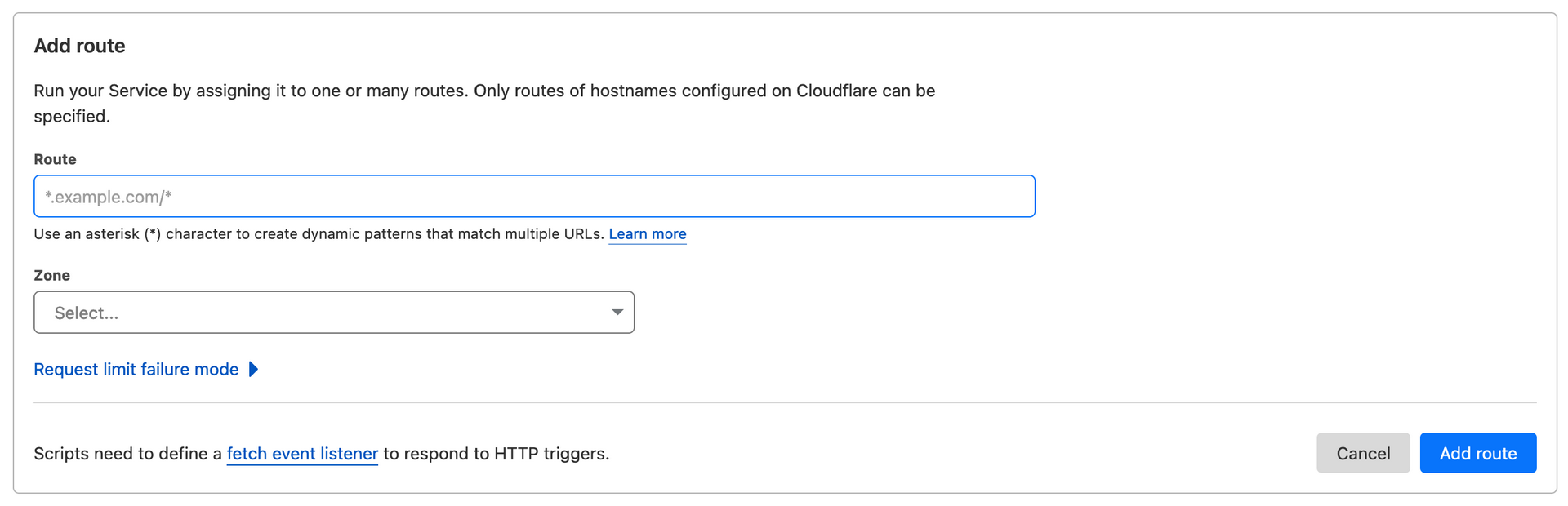
This will make your code live on the Cloudflare edge network, and all incoming requests to this route will be intercepted by the Worker.
Step 4: Test the Allowed Countries
To test that the Worker is correctly allowing requests from the specified countries, try making a request to your API endpoint from an IP address located in one of the allowed countries using a tool like curl
. For example, if your API endpoint is located at https://api.example.com/otp
, you could use the following command to test it:
curl -X POST https://api.example.com/otp
The request should be allowed to proceed if your IP address is located in one of the allowed countries. Otherwise, it should be blocked with a "403 Not allowed" response.
This is a useful way to confirm that your Cloudflare Worker is properly filtering traffic based on the cf-ipcountry
header.
Conclusion
In this tutorial, we showed how to use Cloudflare Workers to allow requests only from specific countries, using the built-in cf-ipcountry
header to efficiently determine the country of the user making the request.
By leveraging the Cloudflare edge network, we can easily allow or block traffic from specific regions without putting extra load on our servers which can be used for a variety of use cases.